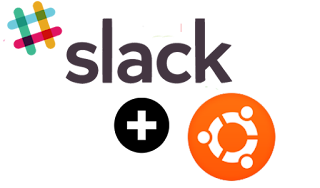
Simple Script
If you don't know
how to set up the webhook integration in Slack check out this video to get you
up to speed.
Also I posted
this tutorial as a youtube video at
Create this
simple script
> sudo vi
/etc/init.d/notify_slack
|
And post the
following into it.
#!/bin/bash
#
# This script notifies slack when the machine is shutdown
or started
#
##############
slack_url=https://hooks.slack.com/services/REPLACE-WITH-YOUR-URL
case "$1" in
start)
curl -H
"Content-type: application/json" \
-X POST -d \
'{
"channel":"#dev_ops",
"text":"Test text Start"
}' $slack_url
;;
stop)
curl -H
"Content-type: application/json" \
-X POST -d \
'{
"channel":"#dev_ops",
"text":"Test text Stop"
}' $slack_url
;;
*)
echo "INFO:
Script used to send notifications to Slack Shutdown/Startup" >&2
exit 3
;;
esac
exit 0
|
Change the
slac_url to your actual webhook url in slack.
Also change the
#dev_ops room to the name of the room you want this posted.
Make it
executable
> sudo chmod
u+x /etc/init.d/notify_slack
|
Now test it.
> sudo
/etc/init.d/notify_slack start
> sudo
/etc/init.d/notify_slack stop
|
It should post this simple message to the slack room.
Register the service
Run the following to register the service so it runs on
startup and shutdown.
> sudo
update-rc.d notify_slack defaults
|
Now reboot!
> sudo reboot
now
|
You should see the stop and stop messages post as the
computer reboots.
Making it prettier
Update your script to the following to make it a bit
prettier.
#!/bin/bash
#
# This script notifies slack when the machine is shutdown
or started
#
##############
slack_url=https://hooks.slack.com/services/REPLACE-WITH-YOUR-URL
case "$1" in
start)
curl -H
"Content-type: application/json" \
-X POST -d \
'{
"channel":"#dev_ops",
"username":"ESXi",
"icon_emoji":":esxi:",
"attachments" : [
{
"fallback":"Server Starting up!",
"color":"good",
"fields" : [
{
"title": "Server Starting up",
"value": "Starting up",
"short":
"true"
}
]
}
]
}' $slack_url
;;
stop)
curl -H
"Content-type: application/json" \
-X POST -d \
'{
"channel":"#dev_ops",
"username":"ESXi",
"icon_emoji":":esxi:",
"attachments" : [
{
"fallback":"Server Shutting down!",
"color":"good",
"fields" : [
{
"title": "Server Shutting down",
"value": "Starting down",
"short": "true"
}
]
}
]
}' $slack_url
;;
*)
echo "INFO:
Script used to send notifications to Slack Shutdown/Startup" >&2
exit 3
;;
esac
exit 0
|
Run a quick test (without rebooting)
> sudo
/etc/init.d/notify_slack start
> sudo
/etc/init.d/notify_slack stop
|
Looks a lot better now.
Note: I uploaded a
custom emoji named :esxi: that is why I get this view when it runs.
Useful information
It may look better but I want some more useful information
to be posted when a machine boots or shuts down. Things like IP address and Disk space left.
Here is what I came up with (You may need to tweak this a
bit)
#!/bin/bash
#
#
#
This script notifies slack
#
when this machine starts up
#
or shuts down
#
########################################
#This
reads in the webhook URL from a file
#just
replace it with you actual webhook URL
slack_url=`cat
/etc/init.d/slack_url`
start_text="Name :
`hostname`"
start_text+="\nIP : `ip route get 8.8.8.8 | awk '{print $NF;
exit}'`"
stop_text+=$start_text
start_text+="\nMemory :
`cat /proc/meminfo | grep MemTotal | awk '{mem= $2/1048576;
printf("%0.2g GiB",
mem)
; exit}'`"
drive_info="\n Drives :"
drive_info="`df
-h | grep Filesystem`"
drive_info+="\n`df
-h | grep /dev/sd
| sed ':a;N;$!ba;s/\n/\\\n/g'`"
case
"$1" in
start)
curl -H
"Content-type:application/json" \
-X POST -d \
'{
"channel":"#dev_ops",
"username" :
"ESXi",
"icon_emoji" :
":esxi:",
"attachments" : [
{
"fallback": "Server
Starting up!",
"color" :
"good",
"fields" : [
{
"title" :
"Server Starting Up!",
"value" :
"'"$start_text"'",
"short" :
"true"
},
{
"title" :
"Drive Status",
"value" :
"'"$drive_info"'",
"short" :
"true"
}
]
}
]
}
' $slack_url
;;
stop)
curl -H
"Content-type:application/json" \
-X POST -d \
'{
"channel":"#dev_ops",
"username" :
"ESXi",
"icon_emoji" :
":esxi:",
"attachments" : [
{
"fallback": "Server
Shutting Down!",
"color" :
"good",
"fields" : [
{
"title" :
"Server Shutting Down!",
"value" :
"'"$stop_text"'",
"short" :
"true"
}
]
}
]
}
' $slack_url
;;
*)
echo "INFO:Script used to send
notifications to Slack Shutdown/Startup" >&2
exit 3
;;
esac
exit
0
|
And here is the results of a reboot.
I get the IP address/Name/Memory size on a startup and Also
a quick view on how much disk space I have left.
This script as is probably won't work on your systems you
will need to tweak it a bit since it is doing a few greps and awks of the
information.
Happy Slacking!
That is nice!
ReplyDelete